Getting Started with Discord Bots using Discord.js
June 08, 2023
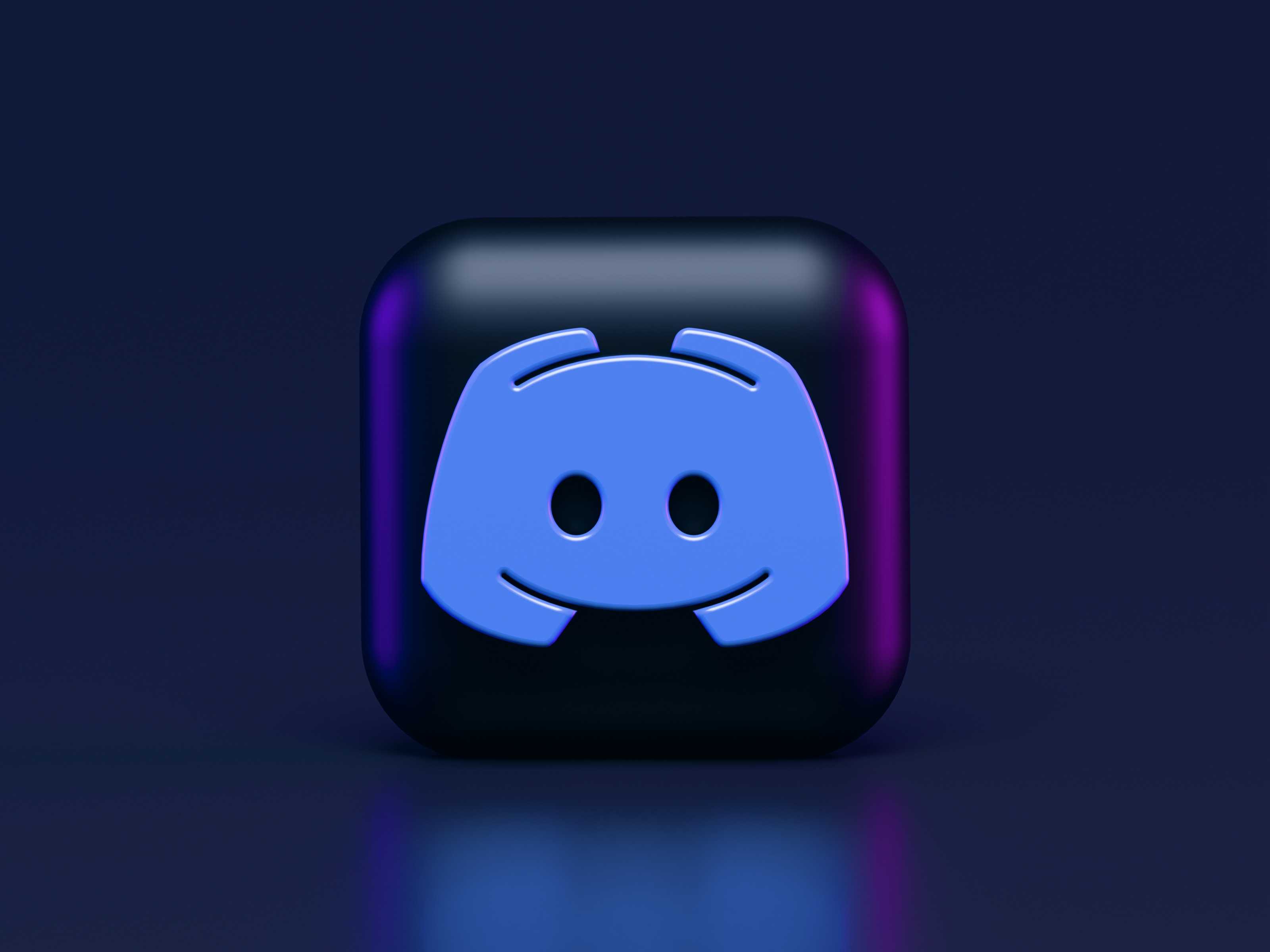
Photo Credit: Alexander Shatov
Introduction
Discord is a popular communication platform among gamers and communities. Discord bots are automated programs that can perform various tasks on Discord servers, such as moderating content, playing music, and much more. In this tutorial, we will be using Discord.js, a powerful Node.js module that allows you to interact with the Discord API.
Prerequisites
Before we get started, make sure you have the following:
- Node.js installed on your computer
- A Discord account
- A Discord server where you have the necessary permissions to add a bot
Setting up the Discord Bot
- Open the Discord Developer Portal and create a new application.
- Give your bot a name and a profile picture.
- Go to the Bot section and click on "Add Bot".
- Set a username for your bot and enable the "Presence Intent" and "Server Members Intent".
- Copy the bot token as we will need it later.
- Go to the OAuth2 section and under the "Scopes" section, select "bot".
- Under the "Bot Permissions" section, select the permissions you want your bot to have on the server.
- Copy the generated OAuth2 URL and paste it into your web browser.
- Follow the prompts to select the server you want to add the bot to.
- Complete the verification process by solving the captcha.
- Your bot should now be added to the selected server.
Creating a Node.js Project
- Create a new folder for your project and navigate to it in the terminal.
- Initialize a new Node.js project by running
npm init
and following the prompts. - Install Discord.js by running
npm install discord.js
.
Writing the Code
- Create a new file called
index.js
. - Require the Discord.js module by adding the following line to the top of your file:
const { Client, GatewayIntentBits } = require("discord.js");
- Create a new instance of the Discord client by adding the following line:
const client = new Client({ intents: [ GatewayIntentBits.Guilds, GatewayIntentBits.GuildMessages, GatewayIntentBits.MessageContent, GatewayIntentBits.GuildMembers, GatewayIntentBits.GuildVoiceStates, ], });
- Add an event listener for when the bot is ready:
client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); });
- Log in to the Discord API using the bot token:
-
create a new file called
config.json
add the token that you copied earlier as follows,{ "token": "your-bot-token-goes-here" }
-
after this import the token to
index.js
file by adding the following lineconst config = require("./config.json");
-
and add the following code at end of
index.js
to implement loginclient.login(config.token);
After implementing all the steps index.js
will look like,
const { Client, GatewayIntentBits } = require("discord.js");
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.MessageContent,
GatewayIntentBits.GuildMembers,
GatewayIntentBits.GuildVoiceStates,
],
});
const config = require("./config.json");
client.on("ready", () => {
console.log(`Logged in as ${client.user.tag}!`);
});
client.login(config.token);
- Run the code by running
node index.js
in the terminal.
Congratulations, you have successfully created your first Discord bot using Discord.js! In the next part of this tutorial, we will be exploring how to add more functionality to your bot.